Sections
INTRODUCTION
PLC Controller Communication API via TCP Protocol
This section provides an overview of the API designed for communication between a PLC controllers and
a local server using the TCP protocol. The local server can be a standalone computer
or a virtual server that acts as an intermediary (Broker). Once the data is received by the local server,
it is further transmitted via HTTPS to the main DLB (Data Logistics Server) service.
This setup ensures secure and efficient data exchange, enabling seamless integration between industrial
automation systems and centralized data management platforms. The API is designed to be robust,
scalable, and easy to implement, supporting real-time communication and reliable data transfer.
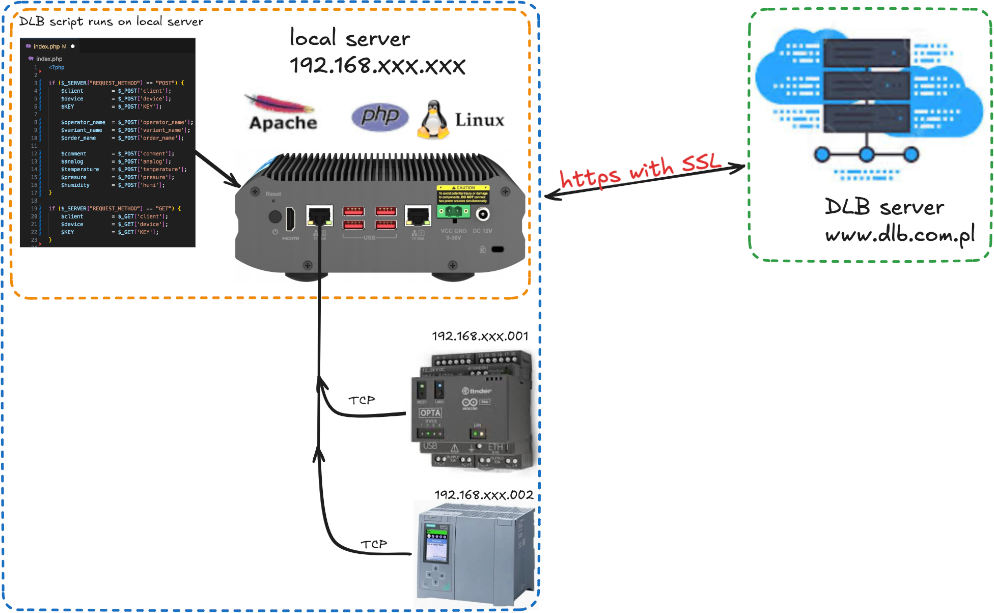
POST request User
NR_IP_SERWERA_LOKALNEGO
$client = 'USER_NAME'; //nazwa zarejestrowanego klienta w systemie DLB
$device = '40:22:D8:93:BE:6D'; //numer MAC loggera
$KEY = '1234567890123456'; //klucz przypisany do loggera
$operator_name = 'pracownik1'; //pracownik
{"result":\"client->lukasz_device->40:22:D8:93:BE:6D_pracownik1__string_ok\"}
POST request Variant
NR_IP_SERWERA_LOKALNEGO
$client = 'USER_NAME'; //nazwa zarejestrowanego klienta w systemie DLB
$device = '40:22:D8:93:BE:6D'; //numer MAC loggera
$KEY = '1234567890123456'; //klucz przypisany do loggera
$variant_name = 'demo2'; //wariant
{"result":\"client->lukasz_device->40:22:D8:93:BE:6D_demo2__string_ok\"}
POST request Order
NR_IP_SERWERA_LOKALNEGO
$client = 'USER_NAME'; //nazwa zarejestrowanego klienta w systemie DLB
$device = '40:22:D8:93:BE:6D'; //numer MAC loggera
$KEY = '1234567890123456'; //klucz przypisany do loggera
$order_name = '123456789'; //zlecenie
{"result":\"client->lukasz_device->40:22:D8:93:BE:6D__123456789__string_ok\"}
POST request Comment
NR_IP_SERWERA_LOKALNEGO
$client = 'USER_NAME'; //nazwa zarejestrowanego klienta w systemie DLB
$device = '40:22:D8:93:BE:6D'; //numer MAC loggera
$KEY = '1234567890123456'; //klucz przypisany do loggera
$comment = 'C1'; //comment from C1 .. C32
{"result":\"client->lukasz_device->40:22:D8:93:BE:6D__123456789__string_ok\"}
POST request string2
NR_IP_SERWERA_LOKALNEGO
$client = 'USER_NAME'; //nazwa zarejestrowanego klienta w systemie DLB
$device = '40:22:D8:93:BE:6D'; //numer MAC loggera
$KEY = '1234567890123456'; //klucz przypisany do loggera
$string2 = 'abcdefghijklm...'; //string, any logs
{"result":\"client->lukasz_device->40:22:D8:93:BE:6D__123456789__string_ok\"}
DLB script
if ($_SERVER["REQUEST_METHOD"] == "POST") {
$client = $_POST['client']; //Nazwa klienta zalogowanego do DLB
$device = $_POST['device']; //numer MAC loggera
$KEY = $_POST['KEY']; //klucz przypisany do loggera
$operator_name = $_POST['operator_name']; //pracownik
$variant_name = $_POST['variant_name']; //wariant
$order_name = $_POST['order_name']; //numer zlecenia
$comment = $_POST['comment'];
$analog = $_POST['analog'];
$temperature = $_POST['temperature'];
$presure = $_POST['presure'];
$humidity = $_POST['humi'];
$string2 = $_POST['string2'];
}
if ($_SERVER["REQUEST_METHOD"] == "GET") {
$client = $_GET['client']; //Nazwa klienta zalogowanego do DLB
$device = $_GET['device']; //numer MAC loggera
$KEY = $_GET['KEY']; //klucz przypisany do loggera
}
// URL, do którego wysyłamy zapytanie
$url = "https://dlb.com.pl/api.php";
//$client = 'lukasz'; //nazwa zarejestrowanego klienta w systemie DLB
//$device = '40:22:D8:93:BE:6D'; //numer MAC loggera
//$KEY = '1234567890123456'; //klucz przypisany do loggera
//$operator_name = 'pracownik1'; //pracownik
//$variant_name = 'demo2'; //wariant
//$order_name = '123456789'; //zlecenie
// Dane, które chcemy wysłać
$data = array(
'login' => $client,
'device' => $device,
'key' => $KEY,
'port' => '7',
'command' => 'STRING',
'user_name' => $operator_name,
'variant' => $variant_name,
'order' => $order_name,
'comment' => $comment,
'analog' => $analog,
'temperature' => $temperature,
'presure' => $presure,
'humidity' => $humidity,
'string2' => $string2
);
// Konwertowanie danych na format URL-encoded
$data_string = http_build_query($data);
// Inicjalizacja CURL
$ch = curl_init();
// Ustawienie opcji CURL
curl_setopt($ch, CURLOPT_URL, $url); // URL POST
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); // dpowiedź jako wynik
curl_setopt($ch, CURLOPT_POST, true); // POST
curl_setopt($ch, CURLOPT_POSTFIELDS, $data_string); // wysyłanie
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, true); // Weryfikacja SSL
curl_setopt($ch, CURLOPT_SSL_VERIFYHOST, 2); // host SSL weryfikacja
// Wykonanie zapytania
$response = curl_exec($ch);
// Sprawdzanie błędów CURL
if(curl_errno($ch)) {
echo 'Curl error: ' . curl_error($ch);
}
// Zamknięcie CURL
curl_close($ch);
// Wyświetlenie odpowiedzi
echo $response;
Requirements
To install requirements, enter the following commands at the terminal prompt
sudo apt update
sudo apt install apache2
sudo apt install php
sudo apt install curl
sudo apt install php8-curl
Next paste the DLB script into a file /var/www/html/index.php
On the end pleas remove the file /var/www/html/index.html
Warning: The local server must never be exposed with an external IP address. Doing so will make it vulnerable to hacker attacks, potentially leading to unauthorized access and the blocking of the local server.